All Possible Combinations Of 4 Numbers Generator
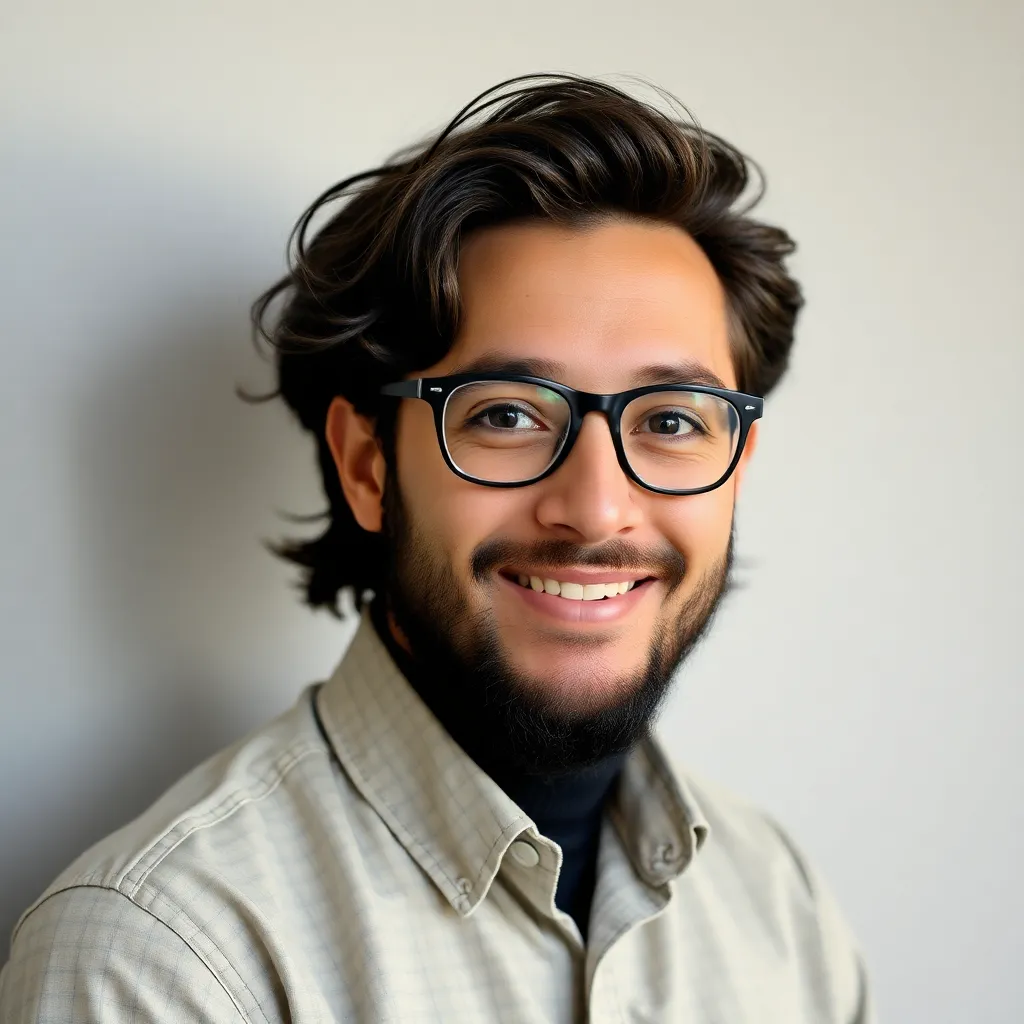
Treneri
Apr 07, 2025 · 5 min read
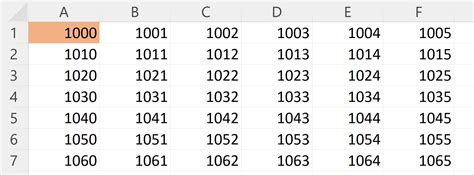
Table of Contents
All Possible Combinations of 4 Numbers Generator: A Comprehensive Guide
Generating all possible combinations of four numbers might seem like a simple task, but the number of possibilities explodes surprisingly quickly, depending on the constraints you impose. This guide delves into the intricacies of generating these combinations, exploring various scenarios, providing illustrative examples, and offering practical solutions for different applications. We'll cover everything from basic combinations without repetition to more complex scenarios with repetition and restrictions.
Understanding the Problem Space
Before diving into generating the combinations, let's understand the scope of the problem. The number of possible combinations depends heavily on two factors:
- The range of numbers: Are we using numbers from 0-9, 1-100, or some other range? A wider range leads to exponentially more combinations.
- Repetition allowed: Are we allowed to use the same number multiple times (e.g., 1123) or must all numbers be unique (e.g., 1234)? Allowing repetition significantly increases the number of possibilities.
Let's analyze the different scenarios:
Scenario 1: Unique Numbers from a Defined Set
This is the most straightforward case. Let's say we're generating combinations of four unique numbers from the set {1, 2, 3, 4, 5, 6, 7, 8, 9, 10}. The number of combinations can be calculated using combinatorics. The formula for combinations without repetition is:
nCr = n! / (r! * (n-r)!)
Where:
- n is the total number of items in the set (10 in our case).
- r is the number of items we're choosing (4 in our case).
- ! denotes the factorial (e.g., 5! = 5 * 4 * 3 * 2 * 1).
Therefore, the number of combinations is 10! / (4! * 6!) = 210.
Generating these combinations programmatically requires algorithms like permutations or backtracking. Many programming languages offer built-in functions or libraries to simplify this. Here's a conceptual Python example (without considering actual implementation details of complex algorithms to keep the article concise and readable):
#Conceptual illustration - requires specific permutation/combination library
import itertools
numbers = range(1, 11)
combinations = list(itertools.combinations(numbers, 4))
print(combinations)
Scenario 2: Numbers with Repetition Allowed
If repetition is allowed, the number of combinations increases drastically. For choosing four numbers from a set of n numbers with repetition, the formula is:
nPr = n^r
Where:
- n is the number of items in the set.
- r is the number of items we're choosing.
Using the same set {1, 2, 3, 4, 5, 6, 7, 8, 9, 10}, and allowing repetition, we get 10^4 = 10,000 possible combinations.
Generating these combinations is simpler than the unique number scenario. Nested loops can effectively achieve this:
#Illustrative Python code, not optimized for extreme scalability
combinations = []
for i in range(1, 11):
for j in range(1, 11):
for k in range(1, 11):
for l in range(1, 11):
combinations.append((i, j, k, l))
print(combinations) #This will be a large list!
Scenario 3: Specific Constraints
Real-world applications often involve additional constraints. For instance:
-
Sum Constraint: Generate all combinations of four numbers that add up to a specific value. This requires a more sophisticated approach, often involving dynamic programming or recursive techniques.
-
Range Constraint: The numbers must fall within a specific range (e.g., between 10 and 50). This can be easily incorporated into the looping approaches mentioned earlier by adjusting the loop bounds.
-
Exclusion Constraint: Certain numbers or combinations are prohibited. This requires adding conditional checks within the generation loops.
-
Ordered vs. Unordered: Are the combinations considered different if the order of numbers changes? (e.g., 1234 and 4321). If order matters, you're dealing with permutations; if order doesn't matter, you're dealing with combinations. The algorithms and counting techniques will differ significantly.
Algorithmic Approaches
Several algorithms are suitable for generating combinations, depending on the constraints:
-
Backtracking: Effective for generating permutations and combinations without repetition. It explores different possibilities by recursively building up the combinations and backtracking when a constraint is violated.
-
Iterative Methods: Nested loops are suitable for scenarios with repetition. However, for large ranges, they can be inefficient.
-
Lexicographic Ordering: This approach generates combinations in a sorted order, useful when you need to process the combinations systematically.
-
Dynamic Programming: Useful for optimization problems, like finding combinations that satisfy a sum constraint.
Practical Implementation Considerations
When implementing a combination generator, several aspects must be considered:
-
Efficiency: For very large ranges, the number of combinations can become astronomically large. Efficient algorithms and data structures are crucial to avoid exceeding memory limits or causing excessive computation time.
-
Memory Management: Storing all combinations in memory might not be feasible for large problems. Consider generating combinations on demand or using techniques like generators to avoid storing the entire set at once.
-
Scalability: The algorithm should be designed to handle varying input sizes gracefully.
-
Error Handling: Implement robust error handling to gracefully manage invalid inputs or edge cases.
-
Language Choice: The choice of programming language depends on the application's requirements. Languages like Python, with their rich libraries, are often preferred for their conciseness and ease of use in these scenarios. However, for performance-critical applications, lower-level languages like C++ might be considered.
Applications of Four-Number Combination Generators
Combination generators have many real-world applications:
-
Lottery Number Generators: Many lottery systems involve choosing a set of numbers from a larger range. Combination generators can be used to simulate lottery draws or analyze the probabilities of winning.
-
Password Generation: While not directly generating four-number combinations, these algorithms can be adapted to generate more complex passwords incorporating numbers, letters, and symbols.
-
Cryptography: Secure key generation and cryptographic algorithms often rely on the generation of combinations and permutations.
-
Combinatorial Optimization: Solving optimization problems involving choosing the best combination from a large set of possibilities.
-
Simulation and Modeling: Generating random or specific combinations for simulations in various fields like scientific experiments, financial modeling, or game development.
-
Data Analysis: Creating test sets or subsets of data by selecting combinations of data points.
Conclusion
Generating all possible combinations of four numbers is a versatile task with diverse applications. The approach depends heavily on the specific constraints and desired output. By understanding the problem space, choosing appropriate algorithms, and considering practical implementation aspects, you can create efficient and reliable combination generators for your specific needs. Remember that for very large ranges, the sheer number of combinations can pose significant computational challenges. Optimized algorithms and resource-aware programming practices are crucial for effective solutions. This guide provided a comprehensive overview, and further research into specific algorithms and data structures might be necessary depending on the complexity of your particular application.
Latest Posts
Latest Posts
-
How Long Is A Sheep Gestation
Apr 09, 2025
-
How Many Cc Is 8 Ounces
Apr 09, 2025
-
Meters To Yards Time Conversion Swimming
Apr 09, 2025
-
Express The Interval Using Inequality Notation
Apr 09, 2025
-
What Is 5 Of 20 Million
Apr 09, 2025
Related Post
Thank you for visiting our website which covers about All Possible Combinations Of 4 Numbers Generator . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.