Rotate The Point To Within 10
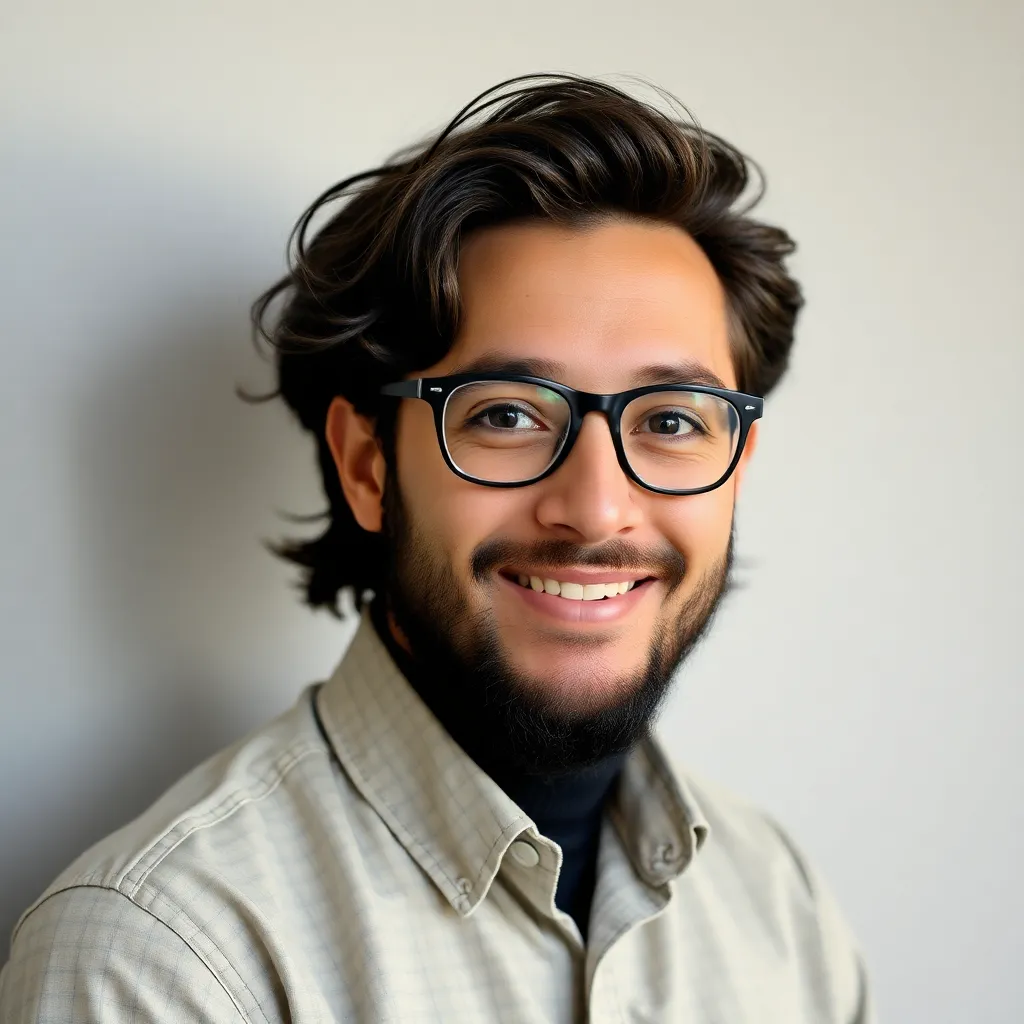
Treneri
May 09, 2025 · 5 min read
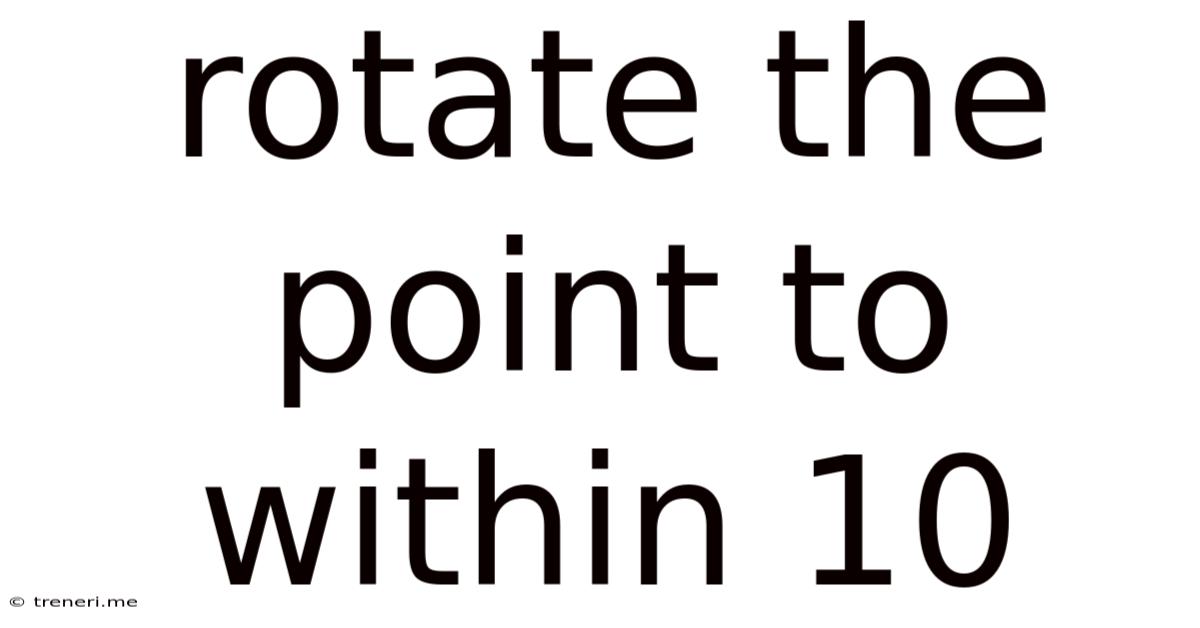
Table of Contents
Rotating a Point to Within a Radius of 10 Units: A Comprehensive Guide
Rotating a point within a specified radius is a fundamental concept in computer graphics, game development, robotics, and various other fields. This article delves into the mathematical principles and practical implementations involved in rotating a point to be within a radius of 10 units. We'll cover different approaches, address potential challenges, and provide illustrative examples to solidify your understanding.
Understanding the Problem
The core challenge lies in transforming the coordinates of a point (x, y) such that its distance from the origin (0, 0) becomes less than or equal to 10 units. This involves two primary steps:
- Calculating the current distance: Determining the initial distance of the point from the origin using the distance formula.
- Rotating and scaling: If the distance exceeds 10, we need to rotate the point by a certain angle and then scale it down to bring it within the desired radius. The choice of rotation angle is often arbitrary, depending on the specific application.
Mathematical Foundation
The process leverages fundamental concepts from coordinate geometry and trigonometry:
1. Distance Formula
The distance (d) between a point (x, y) and the origin (0, 0) is calculated using the Pythagorean theorem:
d = sqrt(x² + y²)
2. Rotation Transformation
Rotating a point (x, y) by an angle θ counter-clockwise around the origin results in a new point (x', y') given by:
x' = x * cos(θ) - y * sin(θ)
y' = x * sin(θ) + y * cos(θ)
3. Scaling Transformation
Scaling a point (x', y') by a factor 's' produces a new point (x'', y''):
x'' = x' * s
y'' = y' * s
Implementation Strategies
We can implement the rotation and scaling using various programming languages and libraries. Let's explore two common approaches:
1. Direct Approach: Scaling to the Radius
This approach directly scales the point to bring its distance to 10 units. It avoids explicit rotation unless a specific rotation is required.
Steps:
-
Calculate the distance: Compute the distance 'd' using the distance formula.
-
Check the distance: If 'd' is less than or equal to 10, no action is needed.
-
Scale the point: If 'd' is greater than 10, scale both x and y coordinates by a factor of 10/d:
x_new = x * (10 / d)
y_new = y * (10 / d)
Code Example (Python):
import math
def scale_to_radius_10(x, y):
d = math.sqrt(x**2 + y**2)
if d <= 10:
return x, y
else:
scale_factor = 10 / d
return x * scale_factor, y * scale_factor
# Example usage:
x, y = 15, 20
x_new, y_new = scale_to_radius_10(x, y)
print(f"Original point: ({x}, {y})")
print(f"Scaled point: ({x_new:.2f}, {y_new:.2f})")
This method is computationally efficient and suitable when a specific rotation isn't necessary. The point is simply scaled radially inward.
2. Rotation and Scaling Approach: Maintaining Angular Position
This approach maintains the original angle of the point while scaling it to the desired radius. This is useful in scenarios where the angular position relative to the origin must be preserved.
Steps:
-
Calculate distance and angle: Calculate 'd' using the distance formula. Calculate the angle θ using
θ = arctan(y/x)
. Consider usingatan2(y, x)
for accurate handling of all quadrants. -
Check distance: If 'd' is less than or equal to 10, no action is required.
-
Scale the radius: Set the new distance to 10.
-
Calculate new coordinates: Use the new distance (10) and the original angle θ to calculate the new coordinates using:
x_new = 10 * cos(θ)
y_new = 10 * sin(θ)
Code Example (Python):
import math
def rotate_scale_to_radius_10(x, y):
d = math.sqrt(x**2 + y**2)
if d <= 10:
return x, y
else:
theta = math.atan2(y, x)
x_new = 10 * math.cos(theta)
y_new = 10 * math.sin(theta)
return x_new, y_new
# Example usage
x, y = 15, 20
x_new, y_new = rotate_scale_to_radius_10(x,y)
print(f"Original point: ({x}, {y})")
print(f"Rotated and Scaled point: ({x_new:.2f}, {y_new:.2f})")
This method preserves the original angle, ensuring that the rotated point lies on the same radial line.
Handling Edge Cases and Optimizations
Several edge cases require special consideration:
- Origin (0, 0): If the point is at the origin, no operation is needed.
- Division by Zero: The
atan2
function handles division by zero gracefully, but explicit checks might be added for robustness. - Floating-point Precision: Floating-point calculations can introduce small inaccuracies. Consider using appropriate tolerance levels when comparing distances.
- Performance Optimization: For large-scale applications, optimize calculations by pre-computing trigonometric functions or using vectorized operations.
Applications and Extensions
The concept of rotating a point within a specific radius finds applications in various domains:
- Game Development: Constraining character or object movements within a circular area.
- Robotics: Limiting the range of motion of robotic arms.
- Computer Graphics: Creating circular or radial effects.
- Data Visualization: Representing data points within a bounded area.
- Signal Processing: Modifying signal amplitudes within a certain range.
This fundamental technique can be extended to:
- Rotating around an arbitrary point: Instead of the origin, you can rotate around any given point (x_c, y_c). This requires translating the coordinates before rotation and then translating them back.
- Three-dimensional rotations: Extending the concept to three dimensions involves using rotation matrices and quaternions.
- Complex rotations: Incorporating multiple rotations around different axes.
Conclusion
Rotating a point to be within a radius of 10 units involves a combination of mathematical concepts and programming techniques. Understanding the distance formula, rotation transformation, and scaling transformation is crucial. This article has presented two primary approaches, discussed edge cases, and highlighted potential applications. The choice between the direct scaling method and the rotation-scaling method depends on the specific requirements of your application. By mastering these techniques, you'll be well-equipped to handle various geometric transformations in your projects. Remember to always consider efficiency and robustness when implementing these algorithms.
Latest Posts
Latest Posts
-
What Is 20 Percent Of 800 000
May 10, 2025
-
Besides 15 And 1 What Is One Factor Of 15
May 10, 2025
-
How To Convert Foot Pounds Into Inch Pounds
May 10, 2025
-
5 Cu Yd To Cu Ft
May 10, 2025
-
43 98 Rounded To The Nearest Tenth
May 10, 2025
Related Post
Thank you for visiting our website which covers about Rotate The Point To Within 10 . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.